gRPC, which stands for Google Remote Procedure Call, is a high-performance, open-source framework developed by Google. This advanced technology allows a client application to call a method on a server application as if it were a local object. This feature simplifies the process of creating distributed applications and services. In many Remote Procedure Call (RPC) systems, gRPC forms the foundation, revolving around the concept of defining a service. This includes the specification of methods that can be remotely invoked, along with their parameters and return types.
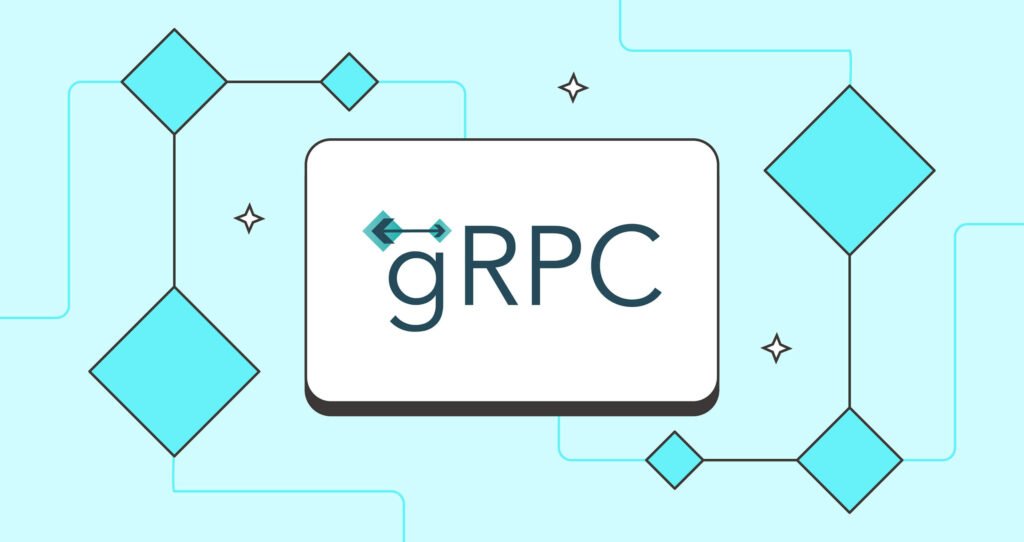
To comprehend this, let’s consider two sides of a system – the server-side and the client-side. On the server-side, the server puts into action this interface and commences a gRPC server to manage client calls. On the flip side, the client-side has a stub that offers identical methods as the server.
One of the significant advantages of gRPC is its versatility and compatibility. The clients and servers utilizing gRPC can function and interact with each other in a multitude of environments . Moreover, these can be written in any of the languages supported by gRPC. This enables you to seamlessly establish a gRPC server in Java, which can communicate with clients in languages such as Go, Python, or Ruby.
A significant aspect of working with gRPC is the use of Protocol Buffers. By default, gRPC employs Protocol Buffers, a robust open-source mechanism by Google for serializing structured data. Protocol Buffers, often known as protobufs, require you to define the data structure you wish to serialize in a .proto file. This file is a simple text file that structures data as messages, where each message serves as a small logical record of data encompassing a series of name-value pairs known as fields.
Once the data structures are specified, you utilize the protocol buffer compiler, protoc, to generate data access classes in your chosen language from your proto definition. These classes provide straightforward accessors for each field, like name() and set_name(), as well as methods to serialize and parse the entire structure to and from raw bytes.
For instance, if you choose Java as your language, running the compiler on the example above will generate a class called Employee. Now, this class can be used within your application to populate, serialize, and retrieve Employee protocol buffer messages.
1. .proto file (employee.proto):
syntax = "proto3";
// Define the Employee message structure
message Employee {
string name ;
int32 id ;
bool is_full_time ;
}
2. Employee Class (Java Example):
// This class is generated from the Employee message definition in employee.proto
public class Employee {
private String name;
private int id;
private boolean isFullTime;
// Getters and setters for each field (automatically generated)
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public boolean isFullTime() {
return isFullTime;
}
public void setFullTime(boolean fullTime) {
isFullTime = fullTime;
}
}
Explanation of the Link:
- The
.proto
file defines the structure of theEmployee
message. It specifies fields likename
(string),id
(integer), andis_full_time
(boolean). - The
protoc
compiler generates code (like theEmployee
class in Java) based on the.proto
definition. This class has corresponding fields (name
,id
, andis_full_time
) that match the message structure. - When you create an
Employee
object in your application code, you can populate its fields with actual employee data. - This
Employee
object can then be serialized into a format suitable for gRPC communication (using generated gRPC client code). This serialized data adheres to the structure defined in the.proto
file. - On the receiving end, the gRPC server deserializes the data back into an
Employee
object (using generated server code), allowing you to access the employee information within your service.
Pseudocode for gRPC Service (greeter.proto):
syntax = "proto3";
service GreeterService {
// RPC method to send greetings with an employee object
rpc SayHelloWithEmployee (HelloRequest) returns (HelloReply) {}
}
message HelloRequest {
// Include the Employee message here (nesting or importing)
Employee employee = 1;
string message = 2; // Optional message to greet the employee
}
message HelloReply {
string greeting = 1;
}
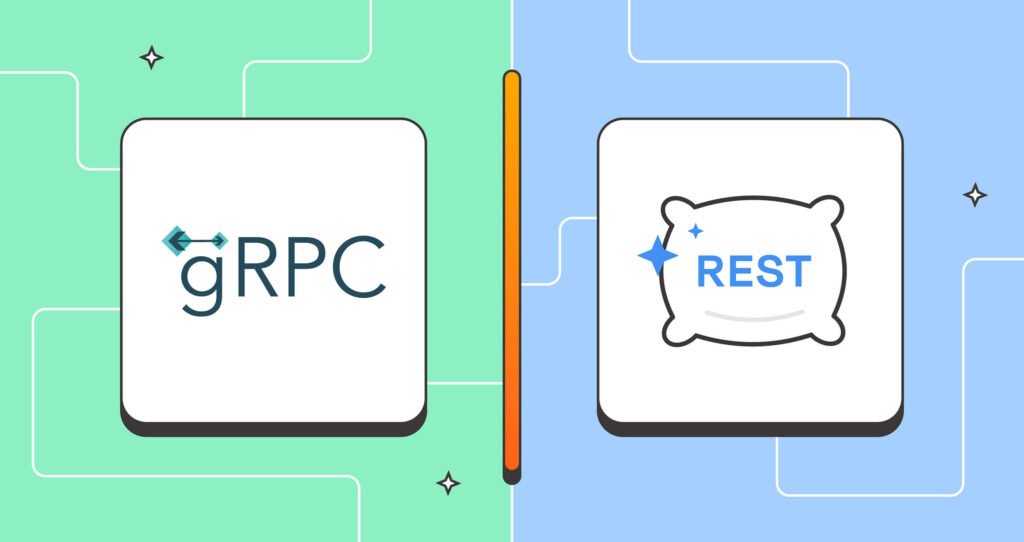
gRPC vs REST
As a software engineer, I often encounter questions about gRPC and REST, especially when it comes to performance. Both gRPC and REST are popular choices for building APIs and services, and each has its strengths and weaknesses.
- Protocol: gRPC uses HTTP/2 for transport which is binary and fully duplex, unlike REST which uses HTTP/1.x, which is text-based and half-duplex. (Click here to read about HTTP/2)
- Data Format: gRPC uses Protocol Buffers (protobuf), a binary format, as its Interface Definition Language (IDL) and to serialize structured data. REST typically uses JSON, which is text-based.
- API Design: REST follows a resource-oriented design, with its standard methods like GET, POST, PUT, DELETE. gRPC, on the other hand, is function-oriented, you define service methods (RPCs) and their request/response types.
- Streaming: gRPC supports 4 types of streaming: Unary, Server-side, Client-side, and Bi-directional. REST, in contrast, does not natively support streaming.
Choosing between gRPC and REST depends on your specific use case:
Conclusion
gRPC offers a compelling alternative to REST for building high-performance, scalable APIs and distributed systems. Its key advantages lie in its efficient use of HTTP/2, compact Protocol Buffers for data serialization, and support for various streaming functionalities.
If you prioritize performance, strong typing, and efficient data exchange within your microservices or internal APIs, gRPC is an excellent choice. However, for public APIs or scenarios requiring broader browser compatibility, REST remains a solid option due to its simplicity and wider adoption.
Ultimately, the decision between gRPC and REST depends on your specific project requirements and priorities. By understanding the strengths and weaknesses of each approach, you can make an informed decision to build robust and performant applications.